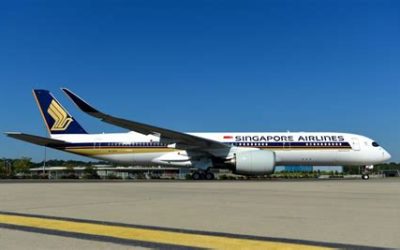
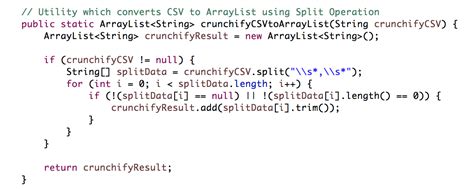
Java 8: Read CSV File into ArrayList with 8 Powerful Tricks
Introduction
Reading CSV (Comma-Separated Values) files into an ArrayList is a fundamental task in data manipulation. Java 8 offers several enhancements that simplify this process, allowing you to work with CSV data more efficiently. In this comprehensive guide, we’ll explore eight robust techniques to read CSV files into an ArrayList using Java 8.
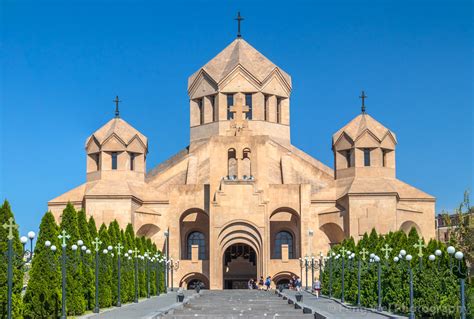
Benefits of Using Java 8 for CSV Parsing
- Simplified Code: Java 8 introduces new stream-based APIs that streamline parsing operations, reducing code complexity.
- Performance Improvements: Stream-based processing allows for parallel execution, improving performance for large CSV files.
- Enhanced Error Handling: Java 8 provides improved exception handling mechanisms, making it easier to handle errors during parsing.
Techniques to Read CSV Files into ArrayList
1. OpenCSV Library
- Third-party library specifically designed for CSV parsing.
- Supports customizing delimiters, skip lines, and reading large files.
CSVReader reader = new CSVReader(new FileReader("data.csv"));
List rows = reader.readAll();
ArrayList> records = new ArrayList<>();
for (String[] row : rows) {
records.add(new ArrayList<>(Arrays.asList(row)));
}
2. Stream and Files API
- Built-in stream-based API introduced in Java 8.
- Provides efficient and flexible ways to read and process CSV data.
Path path = Paths.get("data.csv");
List lines = Files.readAllLines(path);
ArrayList> records = new ArrayList<>();
for (String line : lines) {
String[] values = line.split(",");
records.add(new ArrayList<>(Arrays.asList(values)));
}
3. Scanner Class
- Standard Java class for tokenized input parsing.
- Can be used to parse CSV files by specifying a delimiter.
Scanner scanner = new Scanner(new File("data.csv"));
scanner.useDelimiter(",");
ArrayList> records = new ArrayList<>();
while (scanner.hasNext()) {
ArrayList row = new ArrayList<>();
while (scanner.hasNext()) {
row.add(scanner.next());
}
records.add(row);
}
4. BufferedReader and StringTokenizer
- BufferedReader for line-by-line reading and StringTokenizer for tokenization.
- Efficient and lightweight approach for small to medium-sized CSV files.
BufferedReader reader = new BufferedReader(new FileReader("data.csv"));
ArrayList> records = new ArrayList<>();
String line;
while ((line = reader.readLine()) != null) {
StringTokenizer tokenizer = new StringTokenizer(line, ",");
ArrayList row = new ArrayList<>();
while (tokenizer.hasMoreTokens()) {
row.add(tokenizer.nextToken());
}
records.add(row);
}
5. FastCSV Library
- Focused on high-performance CSV parsing.
- Offers features like multi-threading and custom format handling.
FastCSVParser parser = new FastCSVParser();
parser.setDelimiters(',');
List rows = parser.parse(new File("data.csv"));
ArrayList> records = new ArrayList<>();
for (FastCSVRow row : rows) {
records.add(new ArrayList<>(Arrays.asList(row.getFields())));
}
6. Super-CSV Library
- Comprehensive CSV parsing library supporting various formats and customizations.
- Supports custom column mappings and flexible data conversions.
ICSVReader reader = new CsvListReader();
reader.setDelimiter(',');
reader.setHeaders(new String[] {"Name", "Age", "City"});
List rows = reader.readAll(new File("data.csv"));
ArrayList> records = new ArrayList<>();
for (String[] row : rows) {
records.add(new ArrayList<>(Arrays.asList(row)));
}
7. Commons CSV Library
- Open-source library from Apache Commons project.
- Provides comprehensive functionality for handling various CSV formats and complex parsing operations.
CSVParser parser = CSVParser.parse(new File("data.csv"), StandardCharsets.UTF_8, CSVFormat.DEFAULT);
for (CSVRecord record : parser) {
ArrayList row = new ArrayList<>();
for (String field : record) {
row.add(field);
}
records.add(row);
}
8. Guava CSV Library
- Part of the Google Guava library, focused on efficiency and conciseness.
- Provides a simple and compact API for CSV parsing.
Table table = CsvReader.
read(new File("data.csv"), StandardCharsets.UTF_8);
ArrayList> records = new ArrayList<>();
for (Cell cell : table.cellSet()) {
ArrayList row = new ArrayList<>();
row.add(cell.getRowKey());
row.add(cell.getColumnKey());
row.add(cell.getValue());
records.add(row);
}
Comparison Table of Techniques
Technique | Pros | Cons |
---|---|---|
OpenCSV | Specialized library with comprehensive options | Requires dependency |
Stream and Files API | Efficient and flexible stream-based approach | Requires Java 8 or higher |
Scanner Class | Simple and lightweight | Difficult to customize and handle large files |
BufferedReader and StringTokenizer | Efficient for small to medium-sized files | Requires manual tokenization |
FastCSV Library | High-performance parsing | Requires dependency |
Super-CSV Library | Comprehensive functionality and customization | Complicated API |
Commons CSV Library | Extensive features and open-source | Heavy and verbose |
Guava CSV Library | Concise and efficient | Limited functionality |
Conclusion
Java 8 provides a range of powerful techniques to read CSV files into an ArrayList, each with its own advantages and disadvantages. By understanding these techniques and their nuances, developers can choose the most suitable approach for their specific requirements. Whether you’re dealing with small or large CSV files, simple or complex data formats, Java 8’s stream-based APIs and versatile libraries empower you to efficiently parse and process CSV data in your applications.
Frequently Asked Questions
Q: Which technique is the most efficient for large CSV files?
A: Java 8 Stream and Files API or FastCSV Library offer high performance for large CSV files.
Q: Can I use these techniques to read CSV files with custom delimiters?
A: Yes, most libraries and techniques allow you to specify custom delimiters for CSV parsing.
Q: What if I encounter errors while parsing the CSV file?
A: Java 8’s improved exception handling mechanisms make it easier to catch and handle errors during parsing.
Tips for Effective CSV Parsing
- Validate the CSV file structure and data format before parsing to avoid errors.
- Optimize parsing performance by considering the size and complexity of the CSV file.
- Leverage stream-based APIs for efficient parallel processing of large CSV files.
- Consider using libraries such as OpenCSV or Super-CSV for comprehensive CSV parsing capabilities.
- Handle errors gracefully and provide clear error messages to facilitate debugging.
Future Applications of CSV Parsing
The ability to read CSV files into an ArrayList unlocks various possibilities for data-driven applications. Consider the following examples:
- Data Analysis: Parse CSV data for statistical analysis, trend identification, and predictive modeling.
- Machine Learning: Prepare CSV data as input for machine learning algorithms and models.
- Database Integration: Import CSV data into databases for data warehousing and analytics.
- Data Visualization: Create interactive data visualizations from parsed CSV data.
- Data Exchange: Facilitate data exchange between different applications and systems using CSV as a common format.
By mastering the techniques outlined in this guide, developers can harness the power of Java 8 for efficient CSV parsing and unlock new possibilities for data-driven applications.